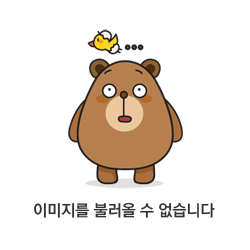
1. Function
(1) function components
Functions are the executable units of code. Functions are usually defined inside a contract, but they can also be defined outside of contracts.
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.7.1 <0.9.0; contract SimpleAuction { function bid() public payable { // Function // ... } } // Helper function defined outside of a contract function helper(uint x) pure returns (uint) { return x * 2; }
Function Calls can happen internally or externally and have different levels of visibility towards other contracts. Functions accept parameters and return variables to pass parameters and values between them.
https://docs.soliditylang.org/en/v0.8.13/structure-of-a-contract.html#functions
function {name} ( {parameters} ) {visibility} {qualifier} {modifier}
- name: 이름이 없는 function은 fallback function
- Parameter: function에 넘겨질 argument들의 이름과 타입
- visibility:
(1) public: 다른 contract나 externally owned account에서 호출 가능
(2) private: 현재 contract에서만 호출 가능
(3) internal: 자신 외에도 자신으로부터 상속받은 contract로부터도 호출 가능
(4) external: public과 유사하나 contract 내로부터 불릴 경우 this keyword를 붙여야
https://docs.soliditylang.org/en/v0.8.13/control-structures.html#function-calls
(2) qualifier
qualifier: function이 contract의 내부 상태, 즉 state variable을 변경할 수 있는 능력
(1) Pure: storage 내의 variable을 읽지 못하고 변경하지 못함
(2) View: 상태 및 storage 내의 variable를 읽을 수는 있으나 변경하지 못함
(3) Payable: 결제 처리 가능. Payable로 선언되지 않은 function에 이더를 보내면 거부.
(3) modifier
Function modifiers can be used to amend the semantics of functions in a declarative way. Overloading, that is, having the same modifier name with different parameters, is not possible. Like functions, modifiers can be overridden.
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.4.22 <0.9.0; contract Purchase { address public seller; modifier onlySeller() { // Modifier require( msg.sender == seller, "Only seller can call this." ); _; } function abort() public view onlySeller { // Modifier usage // ... } }
https://docs.soliditylang.org/en/v0.8.13/structure-of-a-contract.html#function-modifiers
Modifiers can be used to change the behaviour of functions in a declarative way. For example, you can use a modifier to automatically check a condition prior to executing the function. Modifiers are inheritable properties of contracts and may be overridden by derived contracts, but only if they are marked virtual. For details, please see Modifier Overriding.
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.7.1 <0.9.0; contract owned { constructor() { owner = payable(msg.sender); } address payable owner; // This contract only defines a modifier but does not use // it: it will be used in derived contracts. // The function body is inserted where the special symbol // `_;` in the definition of a modifier appears. // This means that if the owner calls this function, the // function is executed and otherwise, an exception is // thrown. modifier onlyOwner { require( msg.sender == owner, "Only owner can call this function." ); _; } }
https://docs.soliditylang.org/en/v0.8.13/contracts.html#modifiers
modifiers: function에 특별한 조건을 추가할 때 사용.
address public owner;
function without_modifier() public {
require(msg.sender == owner)
// function contents
}
function with_modifier() public onlyOwner{
// require(msg.sender == owner)
// function contents
}
modifier onlyOwner(){
require(msg.sender == owner;
_;
}
Modifier도 function처럼 parameter를 받을 수 있고, 특정 조건을 만족하는지를 확인한다. underscore로 표현된 placeholder는, modifier가 적용된 function의 코드가 위치한다. Modifier는 코드의 이해도를 높이고, 조건 검사에 관한 코드를 한 곳에서 관리하므로 유지보수도 용이하다.
[출처] https://docs.soliditylang.org/en/v0.8.13/structure-of-a-contract.html#functions
Structure of a Contract — Solidity 0.8.13 documentation
» Structure of a Contract Edit on GitHub Structure of a Contract Contracts in Solidity are similar to classes in object-oriented languages. Each contract can contain declarations of State Variables, Functions, Function Modifiers, Events, Errors, Struct Ty
docs.soliditylang.org
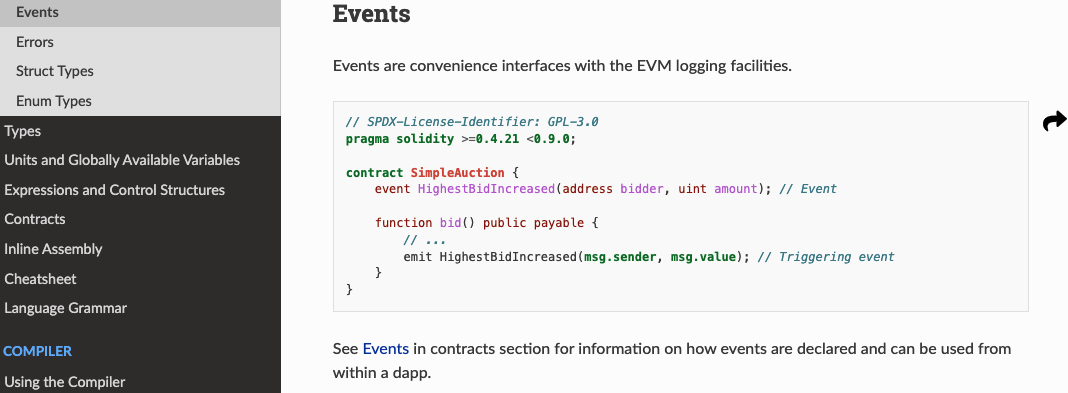
2. events
(1) log
Actually, interacting with any function that costs Gas will generate a txn receipt. There are Gas fees for all functions that save information and change the state of the blockchain. (...) If a transaction gets picked by a miner and that the miner succeeds to mine the block, then the Txn Receipt is generated and added to the block. The block is then broadcast to the network where the other nodes verify it and append it to the chain.
(...) Just like a grocery store receipt, a txn receipt shows you some basic information about what happened, how much it cost, if you hit upon something special ( like an event or a special discount on butter) as well as what functions you interacted with or if you really did get that corned beef has
...the fields are:
- status: true or false — which informs us if the txn was reverted or not — in this case it was true (0x1).
- transaction hash: this is the ID of the transaction
- contract address: where the contract got deployed to
- from: address of the msg.sender
- to: Owner.(constructor) — this is Remix elaborating what is in the Txn Receipt — because in the receipt — when the to address is null— it means that this is the deployment of a new contract.
- gas: This is the gas limit. In Remix, the gas limit is set on the top section of the Deploy & Run Transactions plugin.
- transaction cost: the amount of gas used
- execution cost: this is the code execution portion of the transaction cost
- hash: this is the transaction hash again
- input: this is the encoded input
- decoded input: this is the decoded input parameters. In the contract I deployed, there is no input parameters for the contract’s constructor — so there is nothing here.
decoded output:
- logs: The logs are generated when there is an event in the smart contract. So if there is no event, then there are no logs generated.
- value: the amount of wei sent with this transaction.
Here’s the logged object:
- “from”: is the account I had deployed the contract from.
- “topic” : is the encoded name of the event and its parameters listed by their types.
- “event” : The event that got emitted in the contract when the owner has changed. This event is emitted in the constructor or when interacting with the changeOwner method.
- “args”: is a list of the parameters for the event. The first 2 elements of this array are the parameters listed in the order that they occur and the next 2 elements are the parameters listed by name. There are 2 parameters in the event in the 2_Owner.sol file. So the number of elements in this array depends on how many parameters there are for the event.
The Anatomy of a Transaction Receipt, Rob Stupay, 2020-02-25, Medium
https://medium.com/remix-ide/the-anatomy-of-a-transaction-receipt-d935aacc9fcd
Transaction이 끝나게 되면 transaction receipt가 만들어진다. 다양한 transaction의 결과가 담기는데, transaction 이후의 상태, 사용된 총 gas 양, transaction 동안 생성된 log 등의 정보를 포함한다. 이 log는 블록체인에 기록되며, log를 통해서 contract의 상태가 변경되었다는 것을 외부에 알린다.
(2) events
Events are convenience interfaces with the EVM logging facilities.
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.4.21 <0.9.0; contract SimpleAuction { event HighestBidIncreased(address bidder, uint amount); // Event function bid() public payable { // ... emit HighestBidIncreased(msg.sender, msg.value); // Triggering event } }
https://docs.soliditylang.org/en/v0.8.13/structure-of-a-contract.html#events
Solidity events give an abstraction on top of the EVM’s logging functionality. Applications can subscribe and listen to these events through the RPC interface of an Ethereum client. Events are inheritable members of contracts. When you call them, they cause the arguments to be stored in the transaction’s log - a special data structure in the blockchain. These logs are associated with the address of the contract, are incorporated into the blockchain, and stay there as long as a block is accessible (forever as of now, but this might change with Serenity). The Log and its event data is not accessible from within contracts (not even from the contract that created them).
It is possible to request a Merkle proof for logs, so if an external entity supplies a contract with such a proof, it can check that the log actually exists inside the blockchain. You have to supply block headers because the contract can only see the last 256 block hashes.
You can add the attribute "indexed" to up to three parameters which adds them to a special data structure known as “topics” instead of the data part of the log. A topic can only hold a single word (32 bytes) so if you use a reference type for an indexed argument, the Keccak-256 hash of the value is stored as a topic instead. All parameters without the "indexed" attribute are ABI-encoded into the data part of the log. Topics allow you to search for events, for example when filtering a sequence of blocks for certain events. You can also filter events by the address of the contract that emitted the event. For example, the code below uses the web3.js subscribe("logs") method to filter logs that match a topic with a certain address value:
https://docs.soliditylang.org/en/v0.8.13/contracts.html#events
event: 컨트랙트가 작업을 수행하는 동안 로그를 남길 수 있게 해주는 기능이다.
event는 탈중앙화 어플리케이션과의 연동에도 중요하지만, contract를 디버깅할 때도 유용하게 사용된다.
- event는 contact 내부에서 event keyword로 선언된다.
- event는 argument를 받을 수 있는데, 선언할 때 variable의 이름을 명시할 필요는 없고, 타입만 나열해도 된다. event에게 넘겨진 parameter들은 블록체인 내에 transaction log에 저장된다. argument에 “indexed”라고 선언하면 topics라고 불리는 indexed table에 저장된다.
- event는 함수 안에서 emit 키워드를 통해서 호출된다. 선언했을 때와 같은 타입의 값들을 event로 넘겨지고 이를 블록체인에 저장한다.
[출처] https://docs.soliditylang.org/en/v0.8.13/contracts.html#events
Contracts — Solidity 0.8.13 documentation
Contracts Contracts in Solidity are similar to classes in object-oriented languages. They contain persistent data in state variables, and functions that can modify these variables. Calling a function on a different contract (instance) will perform an EVM f
docs.soliditylang.org
'기술 공부 > 블록체인' 카테고리의 다른 글
스마트 컨트랙트 (5-6) | Solidity(솔리디티) Error Handling (0) | 2022.04.18 |
---|---|
스마트 컨트랙트 (5-5) | Solidity(솔리디티) Contracts, Inheritance, Interface (0) | 2022.04.18 |
스마트 컨트랙트 (5-3) | Solidity(솔리디티) Units and Globally Available Variables (0) | 2022.04.18 |
스마트 컨트랙트 (5-2) | Solidity(솔리디티) State Variable, Type (0) | 2022.04.16 |
스마트 컨트랙트 (5-1) | Solidity(솔리디티) 레이아웃 (0) | 2022.04.14 |